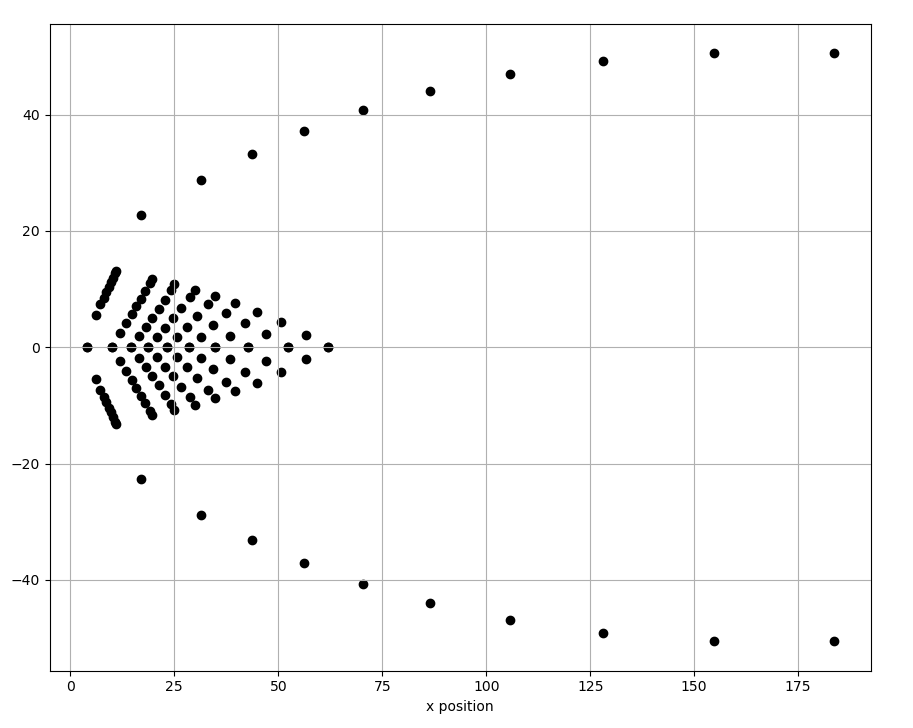
Using Method of Characteristics to Design Nozzle Wall
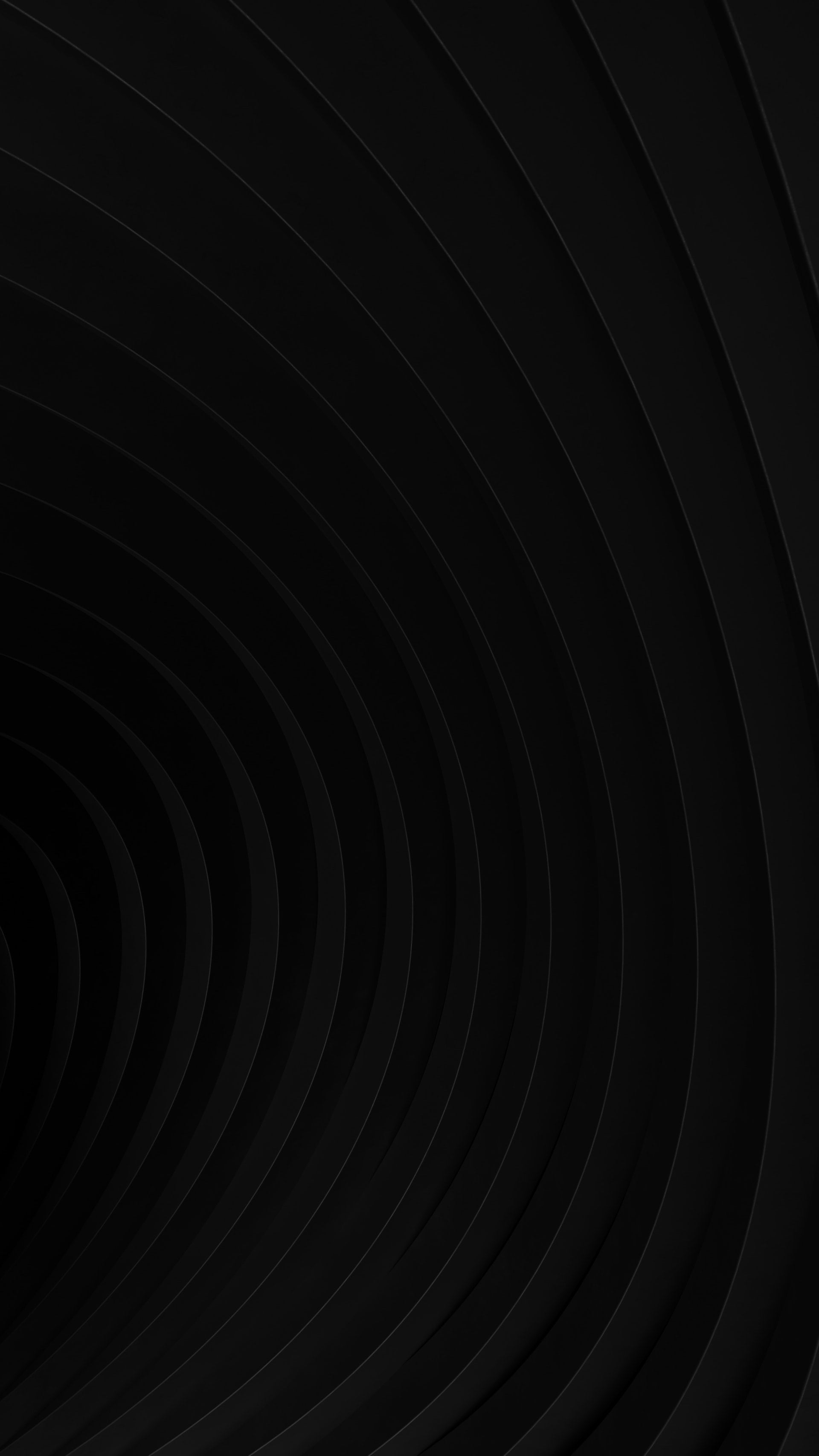
How the code works
1. main.cpp (Entry Point)
- This is the main file that initializes the engine and equations classes, processes command-line arguments, and executes the Method of Characteristics (MOC) computation.
- Reads parameters like the number of lines (-nlines), heat capacity ratio (-gamma), exit Mach number (-exitmach), throat radius (-rthroat), and output file name (-out).
- Calls MOC(Engine, eq) to execute the method of characteristics.
- Writes the results to a file using outputToFile().
2. MOC.cpp (Core MOC Computation)
- Implements the Method of Characteristics (MOC) for supersonic nozzle design.
- Calls Engine.initializePoints() to set up characteristic points.
- Computes Prandtl-Meyer angles using eq.getPMAngle().
- Interpolates angles for characteristic line divisions.
- Initializes points along the wall and centerline.
- Iteratively calculates Mach number, flow angles, and characteristic line intersections.
- Computes xy-coordinates for characteristic points based on previous points.
3. engine.cpp (Engine Class – Manages Points & Flowfield)
- initializePoints():
- Defines the characteristic points (internal points, wall points, and centerline points).
- Assigns flow angles and y-coordinates.
- reset(): Clears stored characteristic points before recomputation.
4. equations.cpp (Mathematical & Thermodynamic Equations)
- returnOutsideTemperatureAndPressure(): Estimates temperature and pressure at different altitudes.
- getExitMach(): Computes exit Mach number from stagnation properties.
- getPMAngle(): Returns Prandtl-Meyer angle.
- getMachFromPM(): Calculates Mach number from Prandtl-Meyer angle using an iterative solver.
- interpolateAngle(): Generates an array of angles between 0 and a given maximum angle.
- returnXYIntersectionPoint(): Finds the intersection of two characteristic lines.
5. cPoint.cpp (Defines Characteristic Point Class)
- Implements the cPoint class, which represents a characteristic point.
- Each point stores:
- Index (m_index)
- Whether it is a wall or centerline point
- Position (m_x, m_y), Mach number, Prandtl-Meyer angle, and flow angle.
6. outputToFile.cpp (Handles Writing Results to File)
- Writes characteristic points to an output file.
- The output includes:
- Index, X-coordinate, Y-coordinate, Mach number, Flow angle, and Prandtl-Meyer angle.
- The output file is later used by the Python script for plotting.
7. PLOTresults.py (Plots the Nozzle Shape)
- Reads the output file (output.txt).
- Extracts X and Y coordinates of characteristic points.
- Plots nozzle contours using matplotlib:
- Plots the top and mirrored bottom profile.
- Allows customizing color and output PNG filename.
8. run_exp.csh (Shell Script)
- Likely an execution script (CSH shell script).
- Typically used to run the main program, set environment variables, or execute multiple runs with different parameters.
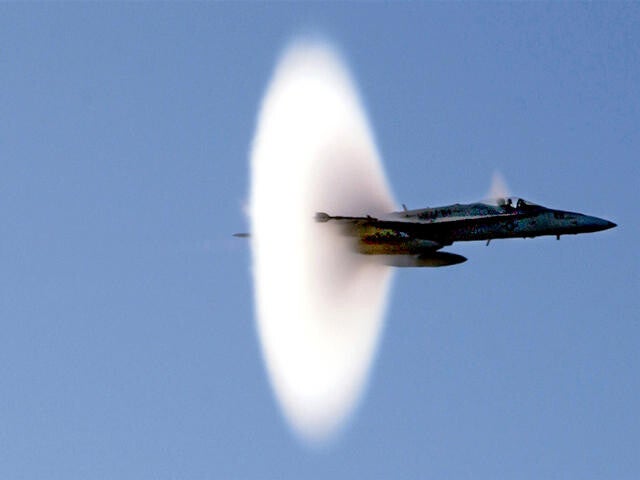
The Method of Characteristics (MOC) is a mathematical technique used to design supersonic rocket nozzles, ensuring smooth, shock-free expansion of exhaust gases to maximize thrust efficiency.
Key Steps in Using MOC for Nozzle Design:
-
Define the Nozzle Exit Conditions:
- Start with the desired exit Mach number based on mission requirements.
- Typically, high expansion ratios are used for optimal exhaust velocity.
-
Generate the Characteristic Mesh:
- Solve the governing equations of gas dynamics, which include Mach number, pressure, temperature, and density variations.
- The method calculates characteristic lines that define the nozzle shape, ensuring a gradual and smooth expansion.
-
Design the Expansion Section:
- A Prandtl-Meyer expansion fan is introduced at the nozzle throat to facilitate smooth supersonic flow expansion.
- The wall contour is shaped using characteristic lines to prevent shocks and minimize losses.
-
Optimize the Nozzle Contour:
- The final diverging section is refined to ensure flow uniformity at the exit, maximizing specific impulse (Isp).
- Adjustments can be made for over-expanded or under-expanded flow depending on altitude conditions.
Using MOC allows engineers to design high-performance, efficient nozzles with minimal flow disturbances, crucial for maximizing thrust in chemical rocket engines. 🚀
Using MOC to design a Nozzle profile
Video tutorial I used
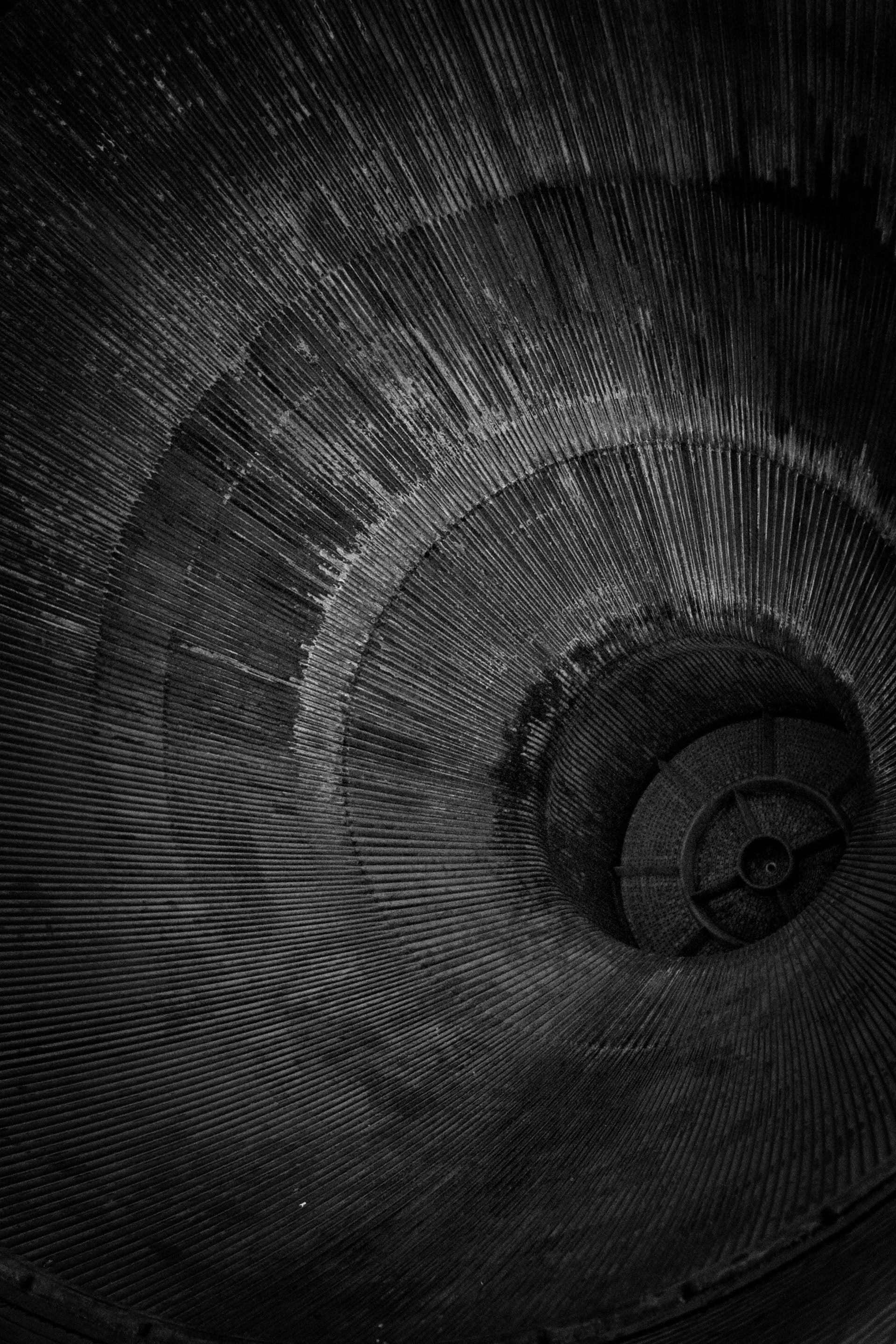
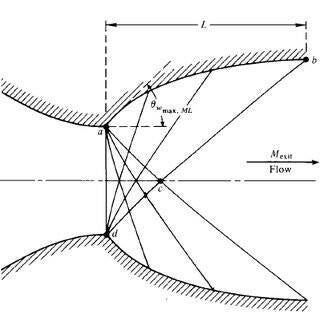